How to Get Values From Object in Java
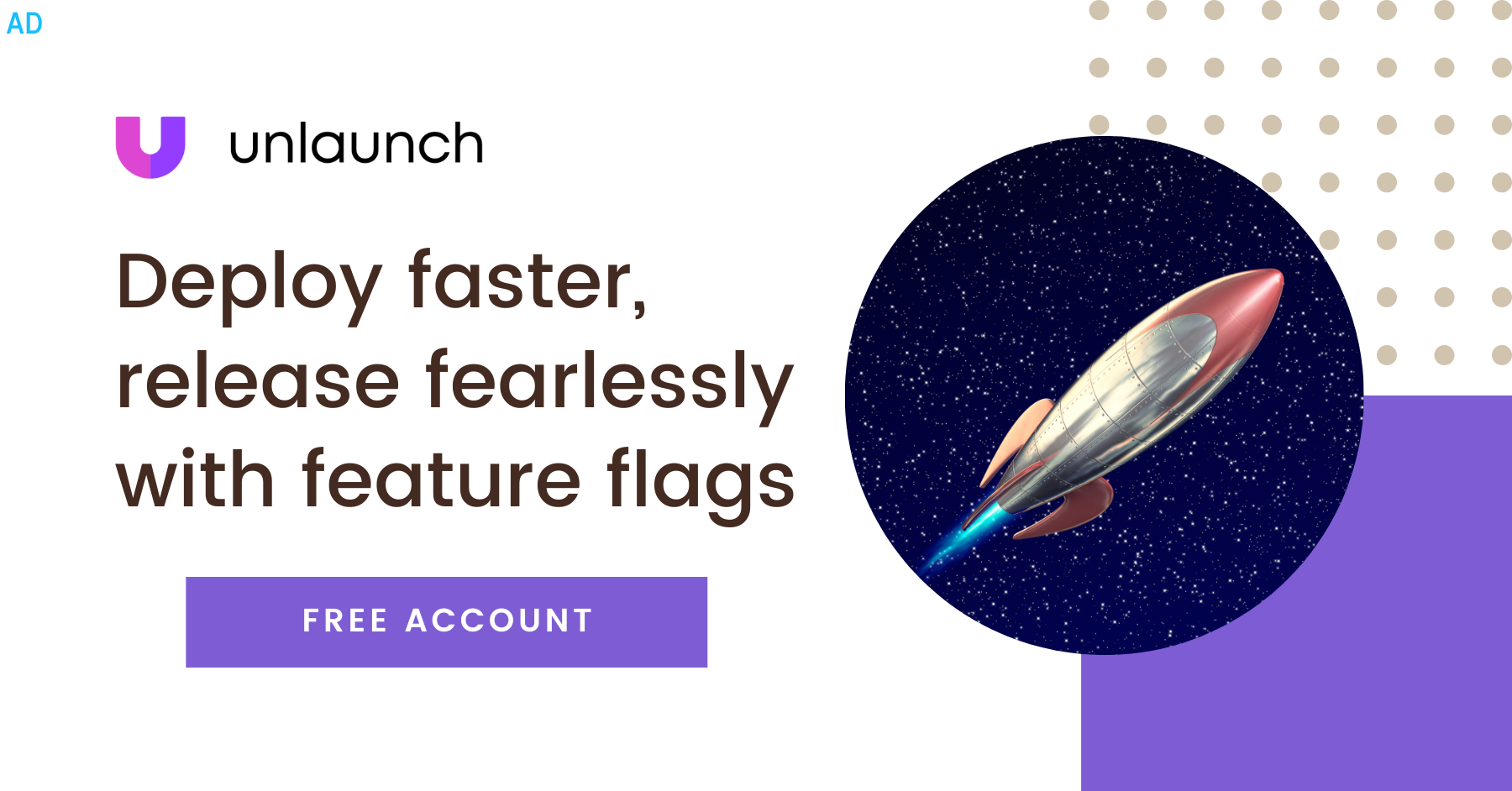
Quick recap: In Java, a Map object is used for mapping keys to values. Duplicate keys are not allowed and each key can have at most one value in a map.
Iterating over keys or values (or both) of a Map object is a pretty common use case and one that developers have to do every so often. Fortunately, the Map interface provides three collection views, which allow a map's contents to be viewed:
- as a set of keys
- collection of values, or,
- set of key-value mappings.
Let's walkthrough some examples and see how to get keys, values or both from a map.
1. Using forEach (Java 8+)
Starting from Java 8, forEach
is easiest and most convenient way to iterate over all keys and values in a map.
map . forEach (( k , v ) -> { System . out . println ( "key: " + k + ", value: " + v ) });
2. Using Map.entrySet() method
Map.entrySet()
method returns a Set
whose entries are key,value pair of the mappings contained in the Map
. This is equivalent to forEach
method descried above.
// iterate over and get keys and values for ( Map . Entry < Integer , String > entry : map . entrySet ()) { Integer k = entry . getKey (); String v = entry . getValue (); System . out . println ( "key: " + k + ", value: " + v ); }
3. Using map.keySet() method - Keys only
Map.keySet() method returns a Set
of keys contained in the map. Use this if you want to obtain the keys only.
Set < Integer > keys = map . keySet (); for ( Integer k : keys ) { System . out . println ( "key: " + k ); }
4. Using map.values() method - Values only
Map.keySet() method returns a Collection
view of the values contained in the map.
Collection < String > values = map . values (); for ( String v : values ) { System . out . println ( "value: " + v ); }
Complete Example
package com.codeahoy.ex ; import java.util.Collection ; import java.util.HashMap ; import java.util.Map ; import java.util.Set ; public class MapExample { public static void main ( String [] args ) { // new map Map < Integer , String > map = new HashMap <>(); // add data map . put ( 1 , "V1" ); map . put ( 5 , "V2" ); map . put ( 10 , "V3" ); System . out . println ( "#1. Using forEach" ); map . forEach ( ( k , v ) -> { System . out . println ( "key: " + k + ", value: " + v ); }); System . out . println ( "#2. Using Map.entrySet() method" ); for ( Map . Entry < Integer , String > entry : map . entrySet ()) { Integer k = entry . getKey (); String v = entry . getValue (); System . out . println ( "key: " + k + ", value: " + v ); } System . out . println ( "#3. Using map.keySet() method - Keys only" ); Set < Integer > keys = map . keySet (); for ( Integer k : keys ) { System . out . println ( "key: " + k ); } System . out . println ( "#4. Using map.values() method - Values only" ); Collection < String > values = map . values (); for ( String v : values ) { System . out . println ( "value: " + v ); } } }
Output:
#1. Using forEach key: 1, value: V1 key: 5, value: V2 key: 10, value: V3 #2. Using Map.entrySet() method key: 1, value: V1 key: 5, value: V2 key: 10, value: V3 #3. Using map.keySet() method - Keys only key: 1 key: 5 key: 10 #4. Using map.values() method - Values only value: V1 value: V2 value: V3
That's all. Hope you enjoyed this post.
If you like this post, please share using the buttons above. It will help CodeAhoy grow and add new content. Thank you!
How to Get Values From Object in Java
Source: https://codeahoy.com/java/How-To-Get-Keys-And-Values-From-Map/
0 Response to "How to Get Values From Object in Java"
Post a Comment